Ruby on Railsのアプリケーションで「Capybara」 + RSpec環境でヘッドレスChromeを使ってE2E(エンドツーエンド)のブラウザテストをするための手順です。ついでにCapybaraのチートシートと、RSpec以外でCapybaraを使う手順を書いておきます。
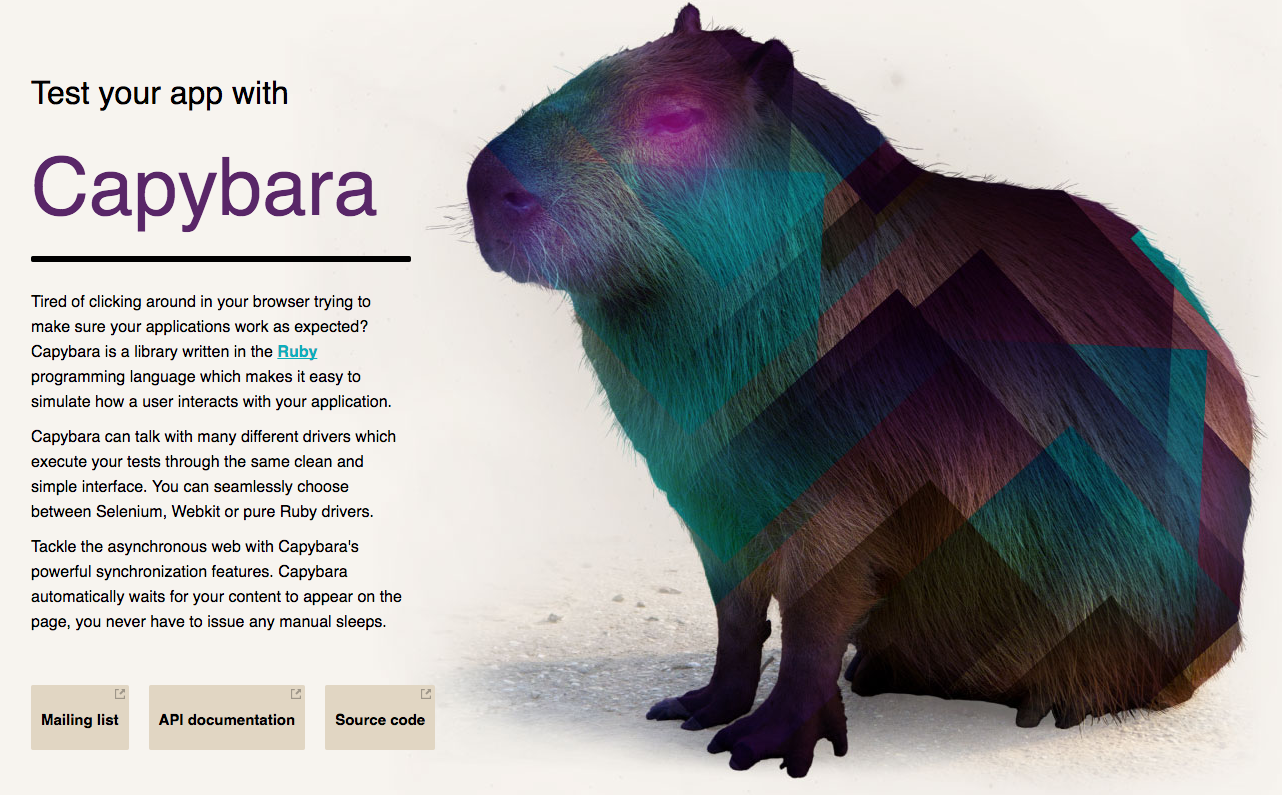
🍄 環境構築
macOSの場合はChrome WebDriverをインストールします。
brew install chromedriver
|
プロジェクト直下のGemfile
に以下を追加して、bundle install
を実行。
group :test do gem 'rspec-rails'
gem 'capybara', require: false gem 'selenium-webdriver', require: false end
|
spec/supports/capybara.rb
を追加して以下を記入。
require 'capybara/rspec' require 'selenium-webdriver'
Capybara.register_driver :chrome do |app| Capybara::Selenium::Driver.new(app, browser: :chrome) end
Capybara.register_driver :headless_chrome do |app| capabilities = Selenium::WebDriver::Remote::Capabilities.chrome( chromeOptions: { args: %w[headless disable-gpu] } )
Capybara::Selenium::Driver.new( app, browser: :chrome, desired_capabilities: capabilities ) end
Capybara.javascript_driver = :headless_chrome
|
rails_helper.rb
にspec/supports/capybara.rb
を読み込む設定を追加。
require 'supports/capybara'
|
😸 テスト
テストは次のように記述します。
require 'rails_helper'
RSpec.describe 'show sell information', type: :feature, js: true do before do end let!(:article) { create(:article) }
scenario 'Go to product pageaaa from top page ' do visit '/' click_on article.title expect(page.current_path).to eq "/articles/#{article.id}" end end
|
これだけでテストの際にヘッドレスのChromeが立ち上がってテストを実施できます。
🎉 Capybaraチートシート
Click系
click_button('button text')
click_link('link text')
click_on('button or link text')
|
フォームの記入
fill_in('some text', with: 'text_tag_selector')
select('some option', with: 'select_tag_selctor')
check('checkbox_selector')
uncheck('checkbox_selector')
choose('yes')
attach_file('attach_file_selector', '/path/to/dog.jpg')
|
要素の検索
find(:css, 'css selector', options)
find(:xpath, 'xpath value', option)
find(:xpath, 'some xpath').set('some text')
find('id_selector', visible: false).text
find_field('id_selector').value
find('checkbox_selector').checked?
find('select_tag_selctor').selected?
find('a', text: 'next').visible?
|
クエリ処理
expect(page).to have_css('#something')
expect(page).to has_xpath('#something')
expect(page).to has_content('#something')
expect(page).to has_no_content('#something')
expect(page).to has_title('#something')
|
スコープの設定
within("//li[@id='example']") do fill_in 'Full Name', with: 'John Due' end
within(:css, "li#example") do fill_in 'Full Name', :with => 'John Due' end
|
その他
Capybara.using_wait_time(30) do expect(find('#message')).to have_text('Complete') end
accept_alert do click_on 'Show Alert' end
dismiss_confirm do click_on 'Delete' end
execute_script 'window.scrollTo(0, 900)'
save_screenshot('xxx.png')
save_and_open_page
|
英語版のCapybaraチートシートは「Ruby Capybara with selenium Cheat Sheat」です。
😎 RSpec以外でのCapybaraの利用
RSpec以外でのCapybaraの利用手順です。
require 'capybara'
Capybara.register_driver :chrome do |app| Capybara::Selenium::Driver.new(app, browser: :chrome) end
Capybara.register_driver :headless_chrome do |app| capabilities = Selenium::WebDriver::Remote::Capabilities.chrome( chromeOptions: { args: %w(headless disable-gpu) } )
Capybara::Selenium::Driver.new( app, browser: :chrome, desired_capabilities: capabilities ) end
Capybara.javascript_driver = :headless_chrome
class CapybaraSampleClass include Capybara::DSL
def sample_method visit 'http://example.selenium.com/' fill_in('name', with: 'sample user') end end
sample = CapybaraSampleClass.new sample.sample_method
|
$ ruby cabybara_sample.rb
|
🐯 参考リンク
🖥 VULTRおすすめ
「VULTR」はVPSサーバのサービスです。日本にリージョンがあり、最安は512MBで2.5ドル/月($0.004/時間)で借りることができます。4GBメモリでも月20ドルです。
最近はVULTRのヘビーユーザーになので、「ここ」から会員登録してもらえるとサービス開発が捗ります!